It is important for developers to be able to easily and quickly test new versions of WooCommerce. The Core Testing Checklist has 30 items in it, and is a manual process. Running through the whole thing is tedious and time consuming, so automating the process would be a big improvement.
End-to-end (e2e) testing tests whether the whole flow of an application is working as expected, and will be an important part in ensuring changes don’t break anything. It automates the manual process of going through all of the different customer-facing and admin-facing pages, performing actions on those pages, and verifying those actions do what is expected.
We have developed an e2e testing suite that should dramatically reduce the time it takes to run through tests before big releases. It works by navigating around a site with ChromeDriver. ChromeDriver simulates a real user by moving the mouse around, clicking on things, and entering input into forms. The results of these actions get compared to the expected results, and the test suite can then determine whether the action was successful. Since this is programmatic, testing can be completed much faster than humanly possible.
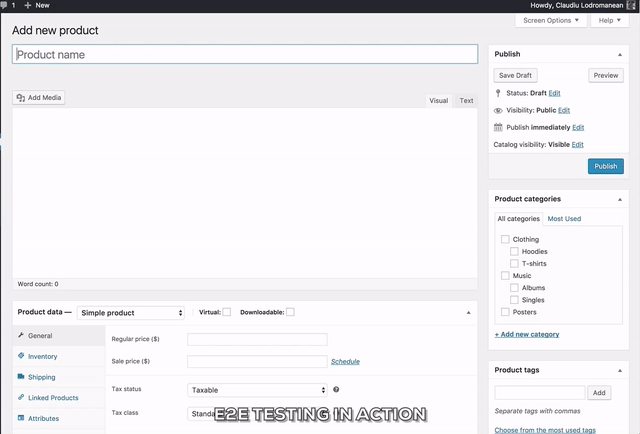
The initial release of the testing suite covers some of our core testing checklist, but not yet all of it. Developers are welcome and encouraged to contribute new tests
Usage
You can use the test suite included in the WooCommerce GitHub repository for testing the base WooCommerce plugin. Follow the Getting Started guide to set up your environment for e2e testing.
You can also use the tests for verifying that an extension didn’t break core WooCommerce functionality. To do this, activate the extension before running the tests. If the tests pass, it will verify that the activated extension did not break functionality covered by the tests.
Please note: this may not work well with some extensions that drastically change the WooCommerce flow or change certain html elements because the tests expect to be able to click on specific elements and have them act in a predetermined way.
Adding end-to-end tests to extensions.
The WooCommerce test suite is built on top of a library we’ve developed for simplifying WooCommerce e2e testing. The library contains a collection of JavaScript objects that can be used for performing common actions on WooCommerce screens, and the objects can be easily extended to add new, custom user actions. You can write test suites for your extensions and dramatically cut down on the amount of time you spend manually testing!
Here is a simple test example using the library:
[code language="javascript"]
// Create a new Shop page object.
const shopPage = new ShopPage(
driver,
{
url: manager.getPageUrl( '/shop' )
}
);
// Click the "Add to Cart" button for "Flying Ninja".
shopPage.addProductToCart( 'Flying Ninja' );
// Create a new Cart page object.
const cartPage = new CartPage(
driver,
{
url: manager.getPageUrl( '/cart' )
}
);
// Verify the cart has the items.
assert.eventually.ok( cartPage.hasItem( 'Flying Ninja' ) );
[/code]
To help you get started with extension e2e testing, we’ve written a helpful tutorial that introduces the library, setting up your environment, writing tests for customer-facing screens, and writing tests for admin-facing screens. Read the tutorial to get started! If you run into any questions about objects or their methods, there is also API documentation.
Contributing
If you encounter any bugs in the library or want to contribute, you can do that here.
Leave a Reply