We have been talking a lot about CRUD since 3.0.0, and the reason is because we want move certain features from the WordPress post database table to new database tables. This improves the performance and scalability of those features. Starting with 3.3.0, our webhooks will not use the posts and comments database tables anymore, and we finally have our first feature migrated to custom tables!
New Webhooks CRUD
Webhooks are a feature that 3rd party developers do not use often or heavily customize, so this make it easy to start moving all of the necessary data for webhooks to a new table.
With CRUD it is now easier to manipulate webhooks too:
// Creating a new webhook.
$webhook = new WC_Webhook();
$webhook->set_user_id( 1 ); // User ID used while generating the webhook payload.
$webhook->set_topic( 'order.created' ); // Event used to trigger a webhook.
$webhook->set_secret( 'secret' ); // Secret to validate webhook when received.
$webhook->set_delivery_url( 'https://webhook-handler.com' ); // URL where webhook should be sent.
$webhook->set_status( 'active' ); // Webhook status.
$webhook->save();
// Updating webhook:
$webhook = wc_get_webhook( $webhook_id );
$webhook->set_status( 'disabled' );
$webhook->save();
// Deleting webhook:
$webhook = wc_get_webhook( $webhook_id );
$webhook->delete( true );
Webhook delivery logs
Delivery logs have been moved from the WordPress comments table to our logging system, which allows more options for handling them instead of having to access the webhook logs in the admin interface.
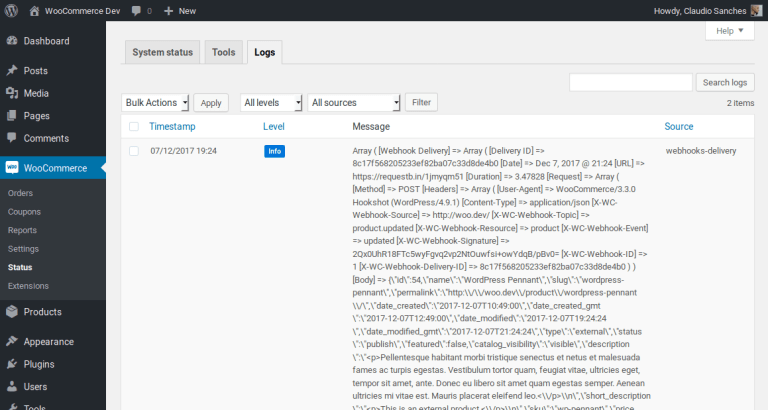
Note: Because of this change all REST API endpoints for webhook delivery logs are now deprecated and will always return as empty. This is because logs now can be saved in the database, as files, or anything else.
Use your favorite message queue tool to process webhooks
It is now possible to write custom code to process webhooks. We used to only have options to deliver a webhook as soon as it is triggered or by WP CRON, but that may not be good for everyone. Until we have a third option with our “event queue” you may want to use Redis, Amazon SQS or something else.
It’s possible to override the delivery like so:
remove_action( 'woocommerce_webhook_process_delivery', 'wc_webhook_process_delivery', 10 );
/**
* Custom process webhook delivery.
*
* @param WC_Webhook $webhook Webhook instance.
* @param array $arg Delivery arguments.
*/
function my_custom_wc_webhook_process_delivery( $webhook, $arg ) {
// Your custom code here.
// Just do not forget to trigger webhook with later:
// $webhook->deliver( $arg )
// Or get the webhook payload as JSON and send with your own delivery method:
// wp_json_encode( $webhook->build_payload( $arg ) )
// Note that custom delivery methods will require extra code to log deliveries with $webhook->log_delivery( $delivery_id, $request, $response, $duration )
}
add_action( 'woocommerce_webhook_process_delivery', 'my_custom_wc_webhook_process_delivery', 10, 2 );
And that’s all folks 👋.
Leave a Reply